BasicX software <--> BX-24
Successful communication relies on devices using the same protocol.
For two devices to successfully talk to each other, they
both need to expect pulses in a certain voiltage range, transmitted
at an exact speed, in a certain order. Data rate is the primary aspect
of a communication protocol. This rate defines the amount of information
per second thats being transmitted (e.g. 9600 bits per second.)
The speed of data transmission is also called baud, and
is usually expressed in kbps, kilobits per second. For example a 56.6k
modem communicates with a computer at 56,600 bits/sec, or 7,075 bytes/sec.
Other components of a serial communication protocol are number of
databits, stop bit, and parity - but these are almost always set the
same so you shouldnt have to worry about them. (8 databits,
1 stop bit, no parity if youre interested.)
Software plays a key role in serial communication, since, if one
of the devices is the computer, it is through software that we most
commonly establish the communication. In our example, the software
will be Macromedia Director. NOTE: the BX is programmed via a serial
cable, through which the program written in the development software
is downloaded to the BX chip. When the BX sends debug statements,
it is sending serial data over the same cable back to the computer.
Therefore, BX software can also be considered as the software interfacing
serially with the chip.
MAKING A SERIAL CABLE
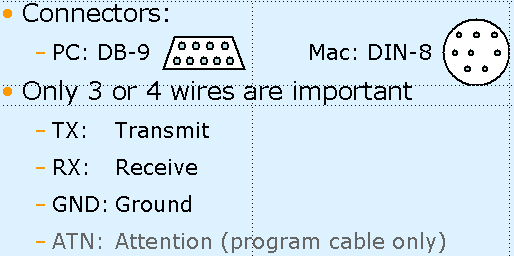
Serial cables can be purchased in most computer stores. There are
both Mac and PC cables, since these two computer platforms have different
cable connectors that need to be used. For a PC, the cable end or
form needed is called DB-9, and the protocol it follows is RS-232.
Mac uses the RS-422 protocol and a DIN-8 connector similar to printer
or modem cables. We will be modifying these standard cables a bit
to get them to work with our microcontroller.
NOTE: If your computer does not have a serial port, but only USB,
you can use a USB serial port adaptor in order to communicate with
the BX-24.
In terms of hardware, the two devices need to be connected via a
serial cable which has three key wires: TX, RX, and GND. In addition
sometimes a fourth signal wire, ATN, is needed (in this case only
for programming). TX stands for Transmit, and its the wire that
sends the data from device A to device B. RX, or Receive, is the wire
for device A to receive data from device B. (Here we will discuss
TX and RX from the microcontrollers point of view.) GND, Ground,
is what creates a common voltage reference level between the two device.
ATN, Attention, is used as a flag for a device to announce
it is ready to transmit and/or receive - it is not needed in a regular
serial cable.
If youre using a PC for your output, you can use your programming
cable as a serial cable - just leave the ATN pin of the cable unconnected.
Remember that TX and RX are usually labeled locally - that is, a
TX pin on device A should connect to the RX pin on device B and vice
versa.
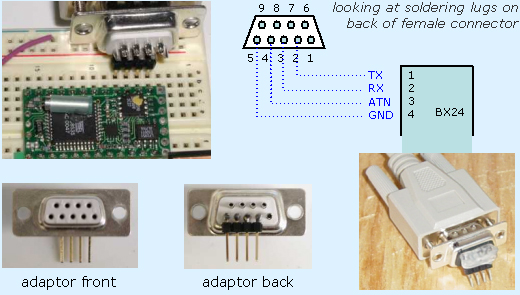
Making a little DB-9 adaptor is the quickest way to make a programming
cable. Simply plug it in to one side of a standard PC serial cable.
Solder right-angle headers to the soldering lugs on the back of a
female DB-9 connector. Youll be connecting pins 2-5 of the serial
cable to pins 1-4 of the BX. If you dont have right-angle headers,
bending straight headers can work. You can also cut off the end of
the serial cable and solder headers directly to the appropriate wires
within (youll have to do this if youre making a Mac serial
cable).
The whitish blob visible in the photo of a serial cable with adaptor
attached is hot glue - this is a great, cheap way to reinforce your
soldering joints and is especially useful for high-stress parts like
cable connectors.
Note: You can only program the BX with the BasicX software on a PC
(although it could be done with some difficulty with a serial-USB
adaptor on a Mac running PC emulation software like VirtualPC).
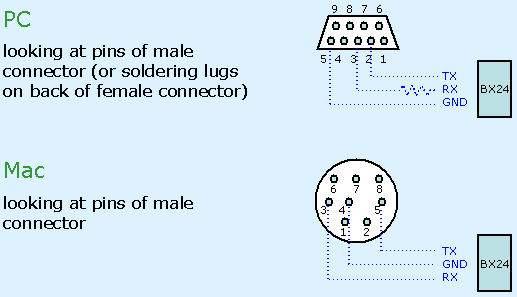
The BX-24 can use any two pins as its serial port. It is easiest
to have these pins be right next to each other. It can also use the
same pins through which you program it, but it is usually recommended
to define different pins for the serial communication keeping the
programming serial port free so that you can reprogram without changing
wiring.
Macs communicate at 9 volts, while PCs use 12V. 9V is close enough
to the 5V the BX expects, but if you are communicating from a PC to
the microcontroller, it is important to use a resistor to limit the
voltage at the receiving pin of the BX. A 22k resistor is standard
but it doesnt need to be exactly that, over 15k should work
(if you are having problems with serial communication you might try
reducing the value of the resistor to less than 22k).
Make sure you have the current limiting resistor in place (if using
a PC) and that the BX-24 is properly grounded before attaching a serial
cable to it.
SERIAL CABLE & THE BREADBOARD
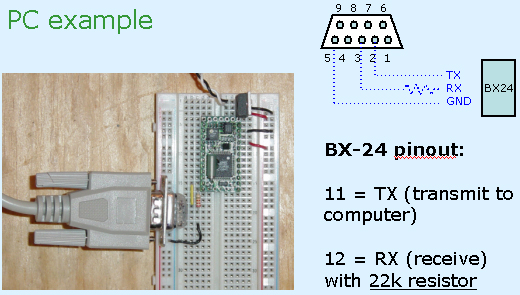
Remember you can use any I/O pins for serial communication, but it
makes sense to use digital-only pins since the ADC pins are limited.
The most common technique used in troubleshooting a cable which is
malfunctioning is the test of continuity. With a multimeter, you can
test if the wire between a pin on both ends of the cable is continuous.
Make sure you know what end of the connector you are looking at when
comparing it to the pin diagrams.
PROGRAM + SERIAL CABLES
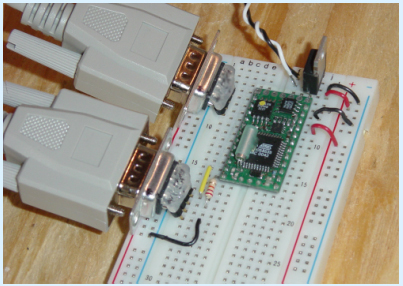
BASICX CODE